推广您的产品
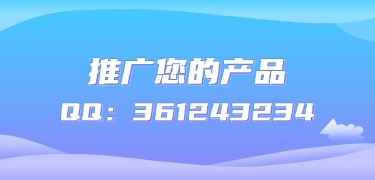
外观
💡 组件名:my-input-code
输入框验证码组件,用于获取邮箱验证码和短信验证码
局部导入
import { defineAsyncComponent } from 'vue'
const MyInputCode = defineAsyncComponent(() => import('/@/components/my-input-code/index.vue'))
const state = reactive({
form: {
email: '',
code: '',
codeId: '',
},
})
//验证邮箱
const validateEmail = (callback: Function) => {
formRef.value.validateField('email', (isValid: boolean) => {
if (!isValid) {
emailRef.value?.focus()
return
}
callback?.()
})
}
//发送验证码
const onSend = (codeId: string) => {
state.form.codeId = codeId
}
<MyInputCode v-model="form.code" :email="form.email" :validate="validateEmail" @send="onSend" />
const state = reactive({
form: {
mobile: '',
code: '',
codeId: '',
},
})
//验证手机号
const validateMobile = (callback: Function) => {
formRef.value.validateField('mobile', (isValid: boolean) => {
if (!isValid) {
phoneRef.value?.focus()
return
}
callback?.()
})
}
//发送验证码
const onSend = (codeId: string) => {
state.form.codeId = codeId
}
<MyInputCode v-model="form.code" :mobile="form.mobile" :validate="validateMobile" @send="onSend" />
参数 | 说明 | 类型 | 默认值 | 可选值 |
---|---|---|---|---|
maxlength | 最大长度 | Number | 6 | - |
seconds | 获取验证码后倒计时,单位秒 | Number | 60 | - |
maxlength | 最大长度 | Number | 6 | - |
startText | 倒计时开始文本 | String | 获取验证码 | - |
changeText | 倒计时进行文本 | String | s秒后重发 | - |
endText | 倒计时结束文本 | String | 重新发送验证码 | - |
mobile | 手机号 | String | - | - |
邮箱 | String | - | - | |
validate | 验证方法 | Function | - | - |